iOS SDK
HotMic offers an iOS SDK so you can let users inside your application watch your streams. This allows you to drive users to your own application, to retain control over your users, unlike in Twitch and Facebook,
To learn more, we recommend looking at our Sample App, which is the fastest way to see the iOS SDK in action inside a sample application.
Then, we recommend reviewing our SDK Installation Guide, found here: iOS SDK Installation Guide
HotMic offers a sample app to see how to use our iOS SDK in your application. Here is a step-by-step guide to downloading and running the HotMic SDK sample applications for iOS.
- A link to the HotMic SDK sample application provided by HotMic.
- An API Key provided by HotMic.
- A JWT token created using a Secret provided by HotMic. Instructions for how to create the token can be found below.
- A white labeled production dashboard where you will produce streams.
For security, when using the SDK your app controls authentication with a JWT Token, which you pass to HotMic.
JSON Web Tokens are an open, industry standard RFC 7519 method for representing claims securely between two parties. - jwt.io
You will receive an API Secret from HotMic, which you will use to create the token. The token will be used to authenticate the user, as well as provide information such as their name, an avatar, a unique ID, or another information which is needed by the SDK.
This is the expected format of the JWT token:
Note, the user_id field should be consistent for each invocation of the SDK for that particular user.
You can also generate JWT tokens online! This is good for test purposes while getting started, or to use the sample app. Here is one way to do it. We do not recommend using this method with any sensitive data.
Go to https://jwt.io/ and scroll down add a payload like the following into the "Payload" section. Paste your secret into the "Verify Signature" section. This screenshot shows how it looks:
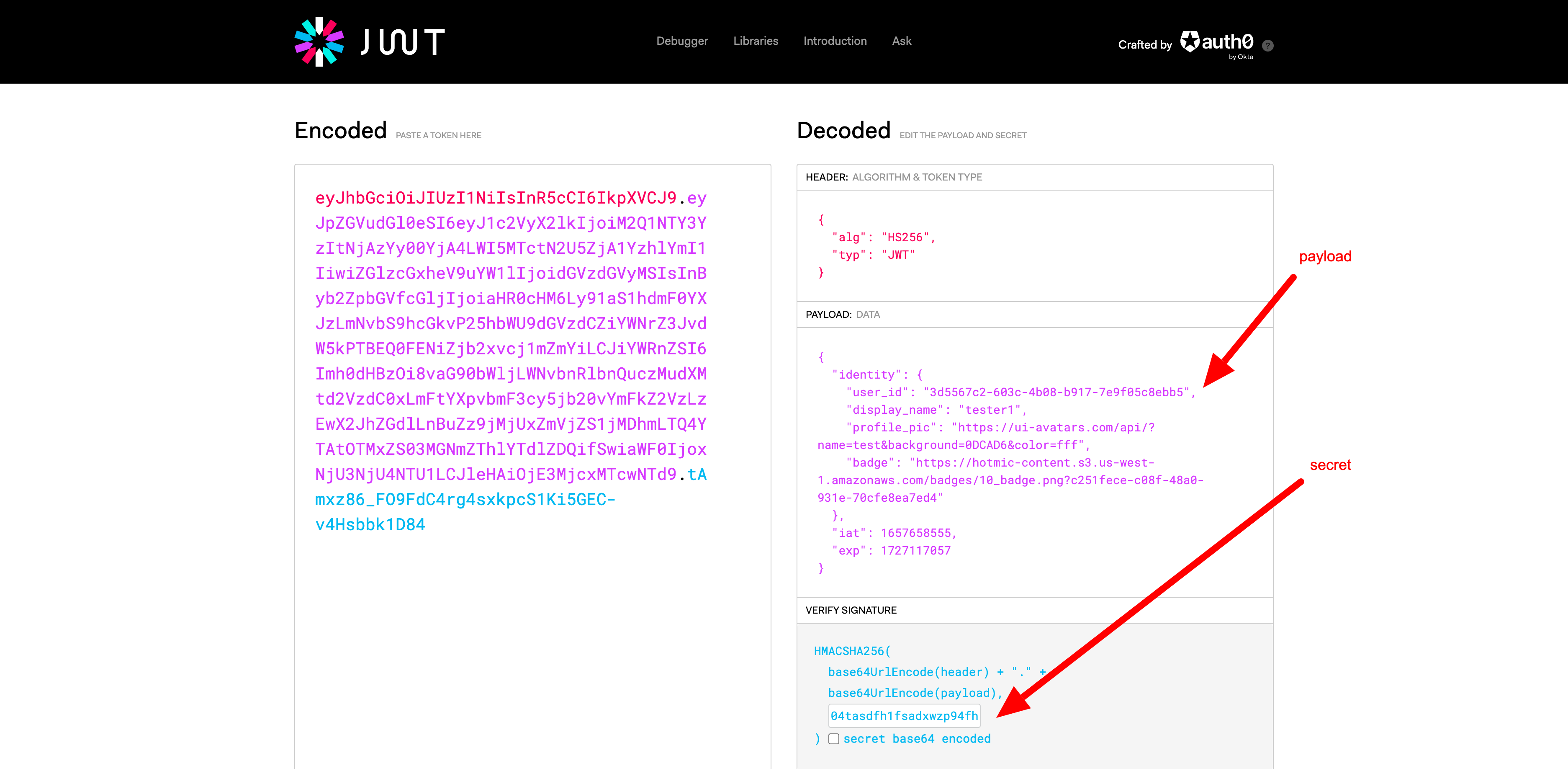
And here is a sample payload that should work for your application:
Then copy the code from the "Encoded" section of this page, and use that in the sample app.
- Unzip the HotMicMediaPlayerSample.zip file, and open MediaPlayerSample.xcworkspace in xCode.
- Open MediaPlayerSample/AppDelegate, and update apiKey and accessToken, inside the call to HMMediaPlayer.initialize.
- Choose your target device or simulator in Xcode, and press Run
- You should see a few streams populate in the app. Tap on one of them to load the media player.
- See the installation guide for a more detailed description of the SDK.
- Use the Production Dashboard to create and start streams. You can use the account [email protected]/password to login, or sign up for a new account.
Here are instructions on how to install the iOS SDK. If you ever have issues installing the iOS SDK, please contact our team.
The name of our SDK is HotMicMediaPlayer. HotMicMediaPlayer allows you to integrate the HotMic player experience into your app. Use this framework to create a HMPlayerViewController for a specific stream and present it full screen.
- API Key and JWT Secret: Will be provided by HotMic.
- iOS 12+
- iPadOS user interface is not tailored to the iPad, its just a larger iPhone view. A future update will expand tablet support.
- macOS apps are not supported.
- Swift UIKit app
- Integration in Objective-C or SwiftUI apps is not officially supported
- App is already live on the App Store
- HotMicMediaPlayer uses the UIWebView framework for YouTube playback. Apple does not allow new apps to use UIWebView. The reason UIWebView is used is due to the fact that WKWebView does not support all features needed for YouTube playback. This component can also be removed if YouTube playback is not required for your use-case.
- App uses view controller based status bar appearance.
- App supports portrait and landscape orientations.
- App disables Bitcode
- Because HotMicMediaPlayer uses ACRCloud which was built without full Bitcode support, HotMicMediaPlayer must disable Bitcode, and your app must as well
Accessibility support including Dynamic Type, VoiceOver, Switch Control, etc
- Default system colors provide high contrast variants
- Dynamic Type support respects the device text size settings
- Large content viewer is supported for small icon buttons
- Views are marked as accessibility elements appropriately
- Accessibility traits are set to identity headings, buttons, selection state, etc
- Accessibility labels are added to ensure controls are titled
- Accessibility hints are added where additional instruction is helpful
When integrating HotMicMediaPlayer into your app, you must disclose the data it and its dependencies collect on the App Store:
- Name - used for product personalization
- Audio data - used for app functionality
- Device ID - used for analytics
- Advertising data - used for developer’s advertising or marketing, analytics, linked to the user’s identity
- Other diagnostics data - used for app functionality, linked to the user’s identity
HotMicMediaPlayer has the following dependencies:
- ACRCloudSDK
- BitmovinPlayer
- TrueTime
- OpenTok
- PubNub
- FittedSheets
- Kingfisher
If your team is already using 1 or more of these dependencies, please contact us so we can ensure to get you the right version of the HotMicMediaPlayer SDK that matches your dependencies.
Add the following to your Podfile, then run pod repo update and then pod install
Add the following keys to your target’s Info tab:
- NSCameraUsageDescription - this is required to join the room with video
- NSMicrophoneUsageDescription - this is required to join the room with audio and sync the stream with a TV
Add the following capabilities to your app’s target:
- Background Modes - Audio, AirPlay, and Picture in Picture
HotMic supports two types of in-app purchases in the player experience: tip the host and join the stream. You can support these in your app as well. You will need to configure these in App Store Connect, then be sure to submit them to Apple for review alongside your app update.
You will need an API key for your app to use the HotMic service. You will also need to create an authorization token. HotMic will provide you with the key and information on how to create the token in the appropriate format.
Initialize HMMediaPlayer with your API key and authorization token. This must be done before any other functions are called in HMMediaPlayer, so we recommend performing initialization in your AppDelegate’s didFinishLaunchingWithOptions. If needed, it can be called again later, for example if the token changes.
A UUID provided by HotMic.
Use any JWT library to create a token. Format is as follows:
Streams are returned in reverse chronological order. You can fetch streams from HotMic with the following filters:
- that are live, scheduled, and/or video-on-demand replays.
- userID: the userid you want to filter the streams by:
- Type: string
- Default: NULL (all possible streams)
- limit: The max number of streams to return
- Type: integer
- Example: 40
- Default: 30
- skip: The number of results to skip you want to not return in the results, typically used for pagination.
- Type: integer
- Example: 10
- Default: 0
Initialize a HMPlayerViewController and present it:
Setting the modalPresentationStyle to a value other than fullScreen is not allowed.
The player view controller is presented in portrait mode, then after presentation it will support rotating to landscape. Note that the player may force rotate to portrait in the experience, for example, if a tall sheet needs to be presented.
Implement the HMPlayerViewControllerDelegateprotocol to support functionality of the player screen.
Each time a player is needed, the following function is called allowing you to provide a custom player that implements the HMPlayerprotocol. Return nilif you'd like to use the default player.
When the player screen will display chat content, the following function is called allowing you to provide a custom chat handler and view controller to display. Provide nilif you'd like to use the default HotMic chat service and UI. Note the handler and view controller can be the same instance of your view controller if it conforms to HMChatHandler.
When the user is finished with the player experience, for example when they tap a button to close the screen, the following function is called allowing you to dismiss it. A PiP view may be provided which allows you to place it into a custom Picture-in-Picture window, such as one provided by the PIPKitlibrary. If you’d like to support PiP, initialize a player view controller with supportsMinimizingToPiP: true, then store this view controller in a strongly held property to prevent it from deinitializing. When you wish to restore the player full-screen, provide the player view to move back into the player view controller then present it again. Be sure to set the view controller property to nilwhen the user wishes to close the player or PiP.
To support returning to the full-screen player experience from Picture-in-Picture, call HMPlayerViewController’s restorePiPView(_:) function and then present the player view controller full screen.
To show a banner ad in the player screen, call HMPlayerViewController’s displayBannerAd(withView:duration:delay:) function. Provide any UIView to display. The view fills the screen width and needs to have a known height such as an intrinsic content size or an Auto Layout constraint that defines a constant height or aspect ratio. If you do not specify a duration of time to display the ad, it will be displayed until you hide it. If you do not specify a delay, it will be displayed immediately. If you do not specify an animationDuration, a default value will be used. If you specify animationDuration: 0, it will not animate.
To remove the banner ad from the player screen, call HMPlayerViewController’s hideBannerAd() function. If you do not specify an animationDuration, a default value will be used. If you specify animationDuration: 0, it will not animate.
To support user profile functionality, you can implement the HMMediaPlayerUserProfileDelegate protocol. If you do not implement this delegate, profile info will be obtained by HotMic rather than your app, and buttons such as follow/unfollow will not be available.
When a user's profile info is to be shown, the following function is called allowing you to provide that user's information. Provide a success result with an HMUserProfile containing information such as their name, profile pic, followers count, following count, if they're following the current user, and if they're followed by the current user. Provide nil for any values that are unavailable. For example, if followingCount is nil the number of people this user follows will not be shown, and if followedByMe is nil the follow/unfollow button will not be shown. Or provide a success result with nil if you would like the profile info to be provided by HotMic rather than your app. Provide a failure result with an Error if one occurred.
The restriction returned should be null or view_and_polls_only, depending on whether the user is restricted.
When the user taps the follow/unfollow button, the following function is called allowing you to record the new following state. Provide an Error to the completion handler if one occurs.
When the user profile is shown, the following function is called allowing you to specify whether the See Full Profile button should be shown.
When the user taps the See Full Profile button, the following function is called allowing you to handle this action, for example by presenting a view controller.
Example of HMUserProfile:
To support tipping hosts and joining their streams for a price, you can implement the HMMediaPlayerInAppPurchaseDelegate protocol and integrate it with your StoreKit in-app purchase code. If you do not implement this delegate, users cannot tip hosts, but can still join the host for free.
When the user opens the tip sheet, the following function will be called to get the SKProducts available to purchase for a host ID. Your app should fetch the products that are applicable to this host from the App Store.
When the user wishes to purchase a tip, the following function will be called. Your app should initiate the in-app purchase process. If the purchase is successful, your app should then submit the tip purchase information including the App Store receipt via HMMediaPlayer’s submitTipPurchase function. HotMic will verify this purchase is legitimate and record the tip if validated. Be sure to provide an error if one occurs in this process, such as if the device cannot make payments, a purchase is already in progress, the transaction was canceled, the transaction failed, failed to get transaction info, no purchase info was found, failed to verify, or failed to process. The completion handler allows you to specify if you want this error’s localizedDescription to be shown to the user and if a button should be provided to retry submitting their purchase information if that request fails. We strongly recommend persisting the purchase information on the device and avoid marking the SKPaymentTransaction finished until the purchase has been successfully submitted, as this allows you to retry submitting the information when StoreKit informs you there is a not-yet-finished purchased transaction.
When the user wishes to retry submitting their purchase information, the following function will be called. Your app should look up the purchase information with the provided product identifier and submit it via HMMediaPlayer’s submitTipPurchase function.
To support join stream in-app purchases, very similar functions as those for tips are available and should be used in the same way.
If you reach out to us we would be happy to provide you with more information and example code from our in-app purchase manager that will allow you to implement this the same way we did.
To be notified of analytics events as they occur throughout the experience, you can implement the HMMediaPlayerAnalyticsEventObserving protocol. Documentation for event names and info keys and values is not yet available.
The HMChatHandlerprotocol defines functions you implement if you'd like to provide a custom view controller for chat while utilizing the HotMic chat service. Your implementation needs to store a reference to an HMChatHandlerDelegateand call its functions to get information and inform the delegate when various events occur.
Store the delegate in a weak optional variable to call its functions in the future.
Store the host ID and use it to indicate when a chat was sent by the host.
Update the configuration of the people feature. Make it accessible in the interface if available. The number of people is provided, allowing you to display this in the interface, for example as a badge on the button.
Update the configuration of the polls feature. Make it accessible in the interface if available. The state of unanswered polls is provided, allowing you to display an indicator in the interface, for example as an "unread" badge on the button.
Update the configuration of the tipping feature. Make it accessible in the interface if available.
Update the top insets of your interface to avoid underlapping other interface elements.
Make the chat input toolbar visible.
Dismiss the chat input toolbar.
Dismiss the keyboard if it is visible.
Handle the backlog of chats and tips to display them in your interface.
Handle new chats, tips, and reactions to insert them into your interface. This function is called periodically to give you the opportunity to handle the pending items. Return true if handled or false if not. If not handled, the same items will be provided in the next invocation.
Remove a chat by ID.
Remove a tip by ID.
Remove a reaction by chat ID, user ID, and type.
The HMChatHandlerDelegateprotocol defines functions your HMChatHandlerimplementation calls to get information and inform HotMic when various events occur.
Asks the delegate if the handler can display the chat input toolbar.
Asks the delegate if the currently authenticated user can moderate another user.
Asks the delegate if the currently authenticated user can make another user a moderator.
Inform the delegate the handler invoked send chat.
Inform the delegate the handler invoked add reaction.
Inform the delegate the handler invoked remove reaction.
Inform the delegate the handler invoked make user moderator.
Inform the delegate the handler invoked report chat.
Inform the delegate the handler invoked report tip.
Inform the delegate the handler invoked block user from chat.
Inform the delegate the handler invoked block user from tip.
Inform the delegate the handler invoked delete chat.
Inform the delegate the handler invoked delete tip.
Inform the delegate the handler invoked view a user's profile.
Inform the delegate the handler invoked the people feature.
Inform the delegate the handler invoked the polls feature.
Inform the delegate the handler invoked the tipping feature.
You can fetch reaction details for a specific chat. Use this to create an interface that lists people who reacted and which reaction they chose if you provide a custom view controller for chat.